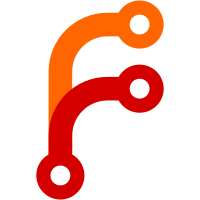
Get event management page almost working! Deleting events works, creating new events works, editing events (almost) works! Just need to figure out how to properly set the date and time fields when editing... Also, user management! You can see a list of users and will theoretically be able to promote officers from the web UI
55 lines
1.5 KiB
Python
55 lines
1.5 KiB
Python
from flask import Flask
|
|
from flask_sqlalchemy import SQLAlchemy
|
|
from flask_migrate import Migrate
|
|
from flask_login import LoginManager
|
|
from flask_bootstrap import Bootstrap5
|
|
from flask_fontawesome import FontAwesome
|
|
|
|
from authlib.integrations.flask_client import OAuth
|
|
|
|
db = SQLAlchemy()
|
|
migrate = Migrate()
|
|
login = LoginManager()
|
|
bootstrap = Bootstrap5()
|
|
font_awesome = FontAwesome()
|
|
oauth = OAuth()
|
|
|
|
def create_app():
|
|
app = Flask(__name__)
|
|
|
|
app.config.from_pyfile('config.py')
|
|
|
|
db.init_app(app)
|
|
migrate.init_app(app, db)
|
|
login.init_app(app)
|
|
bootstrap.init_app(app)
|
|
font_awesome.init_app(app)
|
|
oauth.init_app(app)
|
|
|
|
# register Microsoft Graph sign-in
|
|
tenant = app.config["AZURE_TENANT_ID"]
|
|
AZURE_CLIENT_ID = app.config["AZURE_CLIENT_ID"]
|
|
oauth.register(
|
|
name='azure',
|
|
authorize_url=f"https://login.microsoftonline.com/{tenant}/oauth2/v2.0/authorize",
|
|
access_token_url=f"https://login.microsoftonline.com/{tenant}/oauth2/v2.0/token",
|
|
api_base_url="https://graph.microsoft.com/v1.0/",
|
|
client_kwargs={"scope": "user.read"}
|
|
)
|
|
|
|
from .models import User
|
|
|
|
from .main import bp as main_bp
|
|
app.register_blueprint(main_bp)
|
|
|
|
from .auth import bp as auth_bp
|
|
app.register_blueprint(auth_bp)
|
|
|
|
from .dashboard import bp as dash_bp
|
|
app.register_blueprint(dash_bp)
|
|
|
|
from .admin import bp as admin_bp
|
|
app.register_blueprint(admin_bp)
|
|
|
|
|
|
return app
|