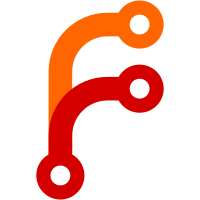
Get event management page almost working! Deleting events works, creating new events works, editing events (almost) works! Just need to figure out how to properly set the date and time fields when editing... Also, user management! You can see a list of users and will theoretically be able to promote officers from the web UI
214 lines
7.7 KiB
HTML
214 lines
7.7 KiB
HTML
{% extends "admin/admin-layout.html" %}
|
|
{% import "bootstrap5/form.html" as wtf %}
|
|
|
|
{% block app_content %}
|
|
<h1>Event list</h1>
|
|
|
|
<table class="table table-striped">
|
|
<thead>
|
|
<tr>
|
|
<th>Name</th>
|
|
<th>Location</th>
|
|
<th>Start Time</th>
|
|
<th>End Time</th>
|
|
<th><button type="button" class="btn btn-primary" data-bs-toggle="modal"
|
|
data-bs-target="#editModal">New</button></th>
|
|
</tr>
|
|
</thead>
|
|
<tbody>
|
|
{% for e in e_list %}
|
|
<tr>
|
|
<td>{{ e.name }}</td>
|
|
<td>{{ e.location }}</td>
|
|
<td>{{ e.start_time }}</td>
|
|
<td>{{ e.end_time }}</td>
|
|
<td>
|
|
<div class="dropdown">
|
|
<a href="#" class="btn btn-primary dropdown-toggle"
|
|
data-bs-toggle="dropdown"><span
|
|
class="caret"></span></a>
|
|
<ul class="dropdown-menu">
|
|
<li class="dropdown-item">
|
|
<a href="#editModal"
|
|
data-bs-toggle="modal" data-id="{{ e.id }}">Edit</a>
|
|
</li>
|
|
<li class="dropdown-item">
|
|
<a href="#deleteModal"
|
|
data-bs-toggle="modal" data-id="{{ e.id }}">Delete Event</a>
|
|
</ul>
|
|
</div>
|
|
</td>
|
|
</tr>
|
|
{% endfor %}
|
|
</tbody>
|
|
</table>
|
|
|
|
<!-- Modals -->
|
|
<div class="modal" id="editModal" tabindex="-1" aria-labelledby="editModalLabel"
|
|
aria-hidden="true">
|
|
<div class="modal-dialog">
|
|
<div class="modal-content">
|
|
<div class="modal-header">
|
|
<h1 class="modal-title fs-5" id="editModalLabel">Event</h1>
|
|
<button type="button" class="btn-close" data-bs-dismiss="modal" aria-label="Close"></button>
|
|
</div>
|
|
<form class="form" role="form" method="post">
|
|
<div class="modal-body">
|
|
{{ form.csrf_token }}
|
|
<div class="form-floating mb-3 required">
|
|
{{ form.name(class="form-control") }}
|
|
{{ form.name.label() }}
|
|
</div>
|
|
<div class="form-floating mb-3">
|
|
{{ form.description(class="form-control") }}
|
|
{{ form.description.label() }}
|
|
</div>
|
|
<div class="form-floating mb-3 required">
|
|
{{ form.location(class="form-control") }}
|
|
{{ form.location.label() }}
|
|
</div>
|
|
<div class="row">
|
|
<div class="col">
|
|
<div class="form-floating mb-3 required">
|
|
{{ form.start_day(class="form-control") }}
|
|
{{ form.start_day.label() }}
|
|
</div>
|
|
</div>
|
|
<div class="col">
|
|
<div class="form-floating mb-3 required">
|
|
{{ form.start_time(class="form-control") }}
|
|
{{ form.start_time.label() }}
|
|
</div>
|
|
</div>
|
|
</div>
|
|
<div class="row">
|
|
<div class="col">
|
|
<div class="form-floating mb-3 required">
|
|
{{ form.end_day(class="form-control") }}
|
|
{{ form.end_day.label() }}
|
|
</div>
|
|
</div>
|
|
<div class="col">
|
|
<div class="form-floating mb-3 required">
|
|
{{ form.end_time(class="form-control") }}
|
|
{{ form.end_time.label() }}
|
|
</div>
|
|
</div>
|
|
</div>
|
|
</div>
|
|
<div class="modal-footer">
|
|
<button type="button" class="btn btn-secondary" data-bs-dismiss="modal">Close</button>
|
|
<button type="submit" class="btn btn-primary">Save changes</button>
|
|
</div>
|
|
</form>
|
|
</div>
|
|
</div>
|
|
</div>
|
|
|
|
<div class="modal" id="deleteModal" tabindex="-1"
|
|
aria-labelledby="deleteModalLabel"
|
|
aria-hidden="true">
|
|
<div class="modal-dialog">
|
|
<div class="modal-content">
|
|
<div class="modal-header">
|
|
<h1 class="modal-title fs-5" id="deleteModalLabel">Delete
|
|
Event?</h1>
|
|
<button type="button" class="btn-close" data-bs-dismiss="modal"
|
|
aria-label="Close">
|
|
</div>
|
|
|
|
<div class="modal-footer">
|
|
<button type="button" class="btn btn-secondary"
|
|
data-bs-dismiss="modal">Cancel</button>
|
|
<button type="button" id="delete" data-bs-dismiss="modal" class=" btn btn-danger">Delete</button>
|
|
</div>
|
|
</div>
|
|
</div>
|
|
</div>
|
|
<script src="{{ url_for('static', filename='js/jquery-3.6.3.min.js') }}" charset="utf-8"></script>
|
|
<script charset="utf-8">
|
|
const deleteButton = document.getElementById("delete")
|
|
|
|
deleteButton.addEventListener("click", (event) => {
|
|
button = $(event.relatedTarget)
|
|
id = deleteButton.dataset.id
|
|
const deleteRequest = new Request(`/admin/event/${id}/delete`)
|
|
|
|
fetch(deleteRequest)
|
|
.then(async (res) => {
|
|
window.alert(await res.text())
|
|
});
|
|
});
|
|
|
|
$('#deleteModal').on('show.bs.modal', function(event) {
|
|
var modal = $(this)
|
|
var button = $(event.relatedTarget)
|
|
var id = button.data("id")
|
|
|
|
// find delete button
|
|
|
|
delButton = document.getElementById("delete")
|
|
delButton.dataset.id = id
|
|
});
|
|
|
|
$('#editModal').on('show.bs.modal', function(event) {
|
|
var modal = $(this)
|
|
|
|
// Zero all fields
|
|
modal.find('#name').val('')
|
|
modal.find('#location').val('')
|
|
modal.find('#description').val('')
|
|
modal.find('#start_day').val('')
|
|
modal.find('#start_time').val('')
|
|
modal.find('#end_day').val('')
|
|
modal.find('#end_time').val('')
|
|
|
|
var button = $(event.relatedTarget)
|
|
var name,description,loc,start_time,start_day,end_time,end_day
|
|
id = button.data('id')
|
|
|
|
if (id) {
|
|
$.get(`/admin/event/${id}`, (data) => {
|
|
console.log(data)
|
|
if (data.status == "error") {
|
|
is_new = "yes"
|
|
} else {
|
|
name = data.name,
|
|
description = data.description,
|
|
loc = data.location
|
|
|
|
start = new Date(data.start_time)
|
|
start_day = start.toLocaleDateString()
|
|
console.log(start_day)
|
|
start_time = start.toLocaleTimeString()
|
|
end = new Date(data.end_time)
|
|
end_day = end.toLocaleDateString()
|
|
end_time = end.toLocaleTimeString()
|
|
}
|
|
|
|
modal.find('#name').val(name)
|
|
modal.find('#location').val(loc)
|
|
modal.find('#description').val(description)
|
|
modal.find('#start_day').val(start_day)
|
|
modal.find('#start_time').val(start_time)
|
|
modal.find('#end_day').val(end_day)
|
|
modal.find('#end_time').val(end_time)
|
|
|
|
|
|
});
|
|
}
|
|
});
|
|
|
|
$('#deleteModal').on('hidden.bs.modal', function(event) {
|
|
location.reload()
|
|
});
|
|
|
|
$('#editModal').on('hidden.bs.modal', function(event) {
|
|
location.reload()
|
|
});
|
|
</script>
|
|
{% endblock app_content %}
|
|
|
|
{% block scripts %}
|
|
{{super()}}
|
|
{% endblock %}
|